Today i want to share how to add a Typing Text animation to your React project using NextJS. When used well this adds interest and personality to your site. We are going to build a fictional home page for a Pastry Twitter Bot like below.
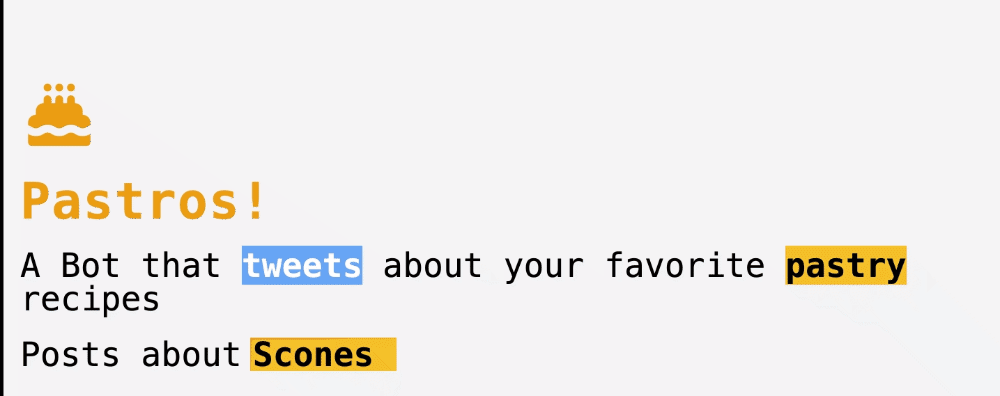
You can find a working example in this sandbox.
Tools we are going to use
- Next.js
- TailwindCSS
- HeroIcons
Setting up your Project
Start by creating a new Next.js project using
create-next-app
. npx create-next-app -e with-tailwindcss next-type-animate cd next-type-animate
This will automatically configure your Tailwind setup based on the Official Next.js example from the Tailwind Guide.
Once done ensure you import TailwindCSS Default Colors as below in your
tailwind.config.js
file.//tailwind.config.js const colors = require('tailwindcss/colors'); module.exports = { ... theme: { colors: { transparent: 'transparent', current: 'currentColor', black: colors.black, white: colors.white, gray: colors.trueGray, yellow: colors.amber, blue:colors.blue } } ... }
Setup Home UI
Install the awesome HeroIcons icon library.
yarn add @heroicons/react
From the
index.js
file add the following code. We import the CakeIcon from the HeroIcons library to give some text. If you need to to get a Tailwind refresher you can check out their documentation.// index.js import { CakeIcon } from '@heroicons/react/solid'; ... <div className="flex flex-col items-start justify-center min-h-screen py-2 bg-gray-100"> <Head> <title>Pastros</title> <link rel="icon" href="/favicon.ico" /> </Head> <main className="px-8"> {/* Header */} <div className="flex flex-col space-y-8 items-start justify-start"> <CakeIcon className="w-28 text-yellow-500" /> <h1 className="font-mono text-7xl font-bold text-yellow-500 tracking-wide"> Pastros! </h1> <h3 className="font-mono text-back text-5xl"> A Bot that{' '} <strong className="bg-blue-400 text-white">tweets</strong> about your favorite <strong className="bg-yellow-400">pastry</strong>{' '} recipes </h3> <div className="font-mono text-5xl flex space-x-3 "> <h4> Posts about </h4> {/* Text to Animate */} <strong className="bg-yellow-400 px-1"> Cakes </strong> </div> </div> </main> </div>
When you run you should achieve this layout.
.jpg?table=block&id=0a2bfd66-8daf-499b-9470-8ae83776ffba&cache=v2)
Adding React-Typical.
React-Typical is a React library built by Catalin Miron that makes displaying typing animation in React easier. It is also lightweight consisting of ~400 bytes of Javascript.
Install React Typical from your project folder.
yarn add react-typical
Import React Typical into your
index.js
file.//index.js import Typical from 'react-typical'
Add the Typical component and replace the 'Cakes' text.
//index.js <strong className="bg-yellow-400 px-1"> <Typical steps={[ 'Cakes', 1000, 'Pies', 1000, 'Biscuits', 1000, 'Scones', 1000, ]} wrapper="p" loop={Infinity} /> </strong>
React-Typical requires the
steps
prop which includes an array of items to be animated in pairs where the first element is Text to be animated and the next is the duration of animation. You can add several sets of data but for decent visual appeal keep the duration to be more than 300ms
(so it doesn't appear like the Flash is typing ⚡️🙂). The wrapper
prop defines what element the text should with then the loop
prop defines how many times the text should entire animation should loop. In our case we've defined it as Infinity
which is a non-number global scope variable that keeps the animation looping.Conclusion
Take a look at the project repo and hit the ⭐️ if you liked it :) https://github.com/DavidAmunga/next-type-animate
If you have any issues or reviews on this project I will be glad if you could open issue on the repo or just message me.